The RudderStack iOS SDK lets you track your customer event data from your iOS applications and send it to your specified destinations via RudderStack.
Refer to the GitHub codebase to get a more hands-on understanding of the SDK.
You can integrate the iOS SDK with your tvOS and watchOS apps and seamlessly track user events without any additional configuration.
The SDK supports tvOS tracking in version 1.1.0 and above. watchOS tracking is supported in version 1.3.1 and above.
A new version of the iOS SDK (v2) is now available. Some key features of this release include:
- Support for tracking events from your macOS apps
- Support for tracking push notifications
For more information, refer to the iOS SDK v2 documentation.
SDK setup requirements
To set up the RudderStack iOS SDK, the following prerequisites must be met:
- You will need to set up a RudderStack account.
- Once signed up, set up an iOS source in the dashboard. For more information, see Adding a source. You should then see a Write Key for this source, as shown below:
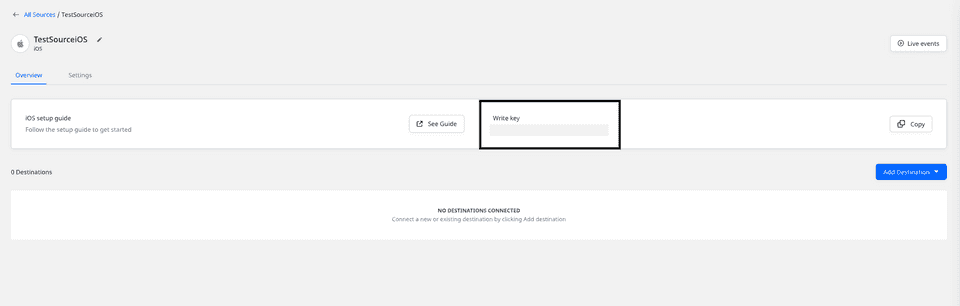
You will also need a data plane URL. Follow this section for more information on the data plane URL and where to get it.
Finally, you will need a Mac with the latest version of Xcode.
Installing the RudderStack iOS SDK
We distribute our iOS SDK through Cocoapods and Carthage. The recommended and easiest way to add the SDK to your project is through Podfile
. Follow these steps:
- Add the RudderStack SDK to your
Podfile
, as shown:pod 'Rudder' - Then, run the following command:pod install
- Add the RudderStack SDK to your
Cartfile
, as shown:github "rudderlabs/rudder-sdk-ios" - Then, run the following command:carthage update
Remember to include the following code in all .m
and .h
files or your .swift
files where you want to refer to or use RudderStack SDK classes.
#import <Rudder/Rudder.h>
import Rudder
RudderStack uses SQLite to store the events before sending them to RudderStack data plane. Making calls like SQLite.shutdown()
which is not thread-safe might lead to unexpected crash.
Initializing the RudderStack client
Put this code in your AppDelegate.m
file under the method didFinishLaunchingWithOptions
:
RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withDataPlaneUrl:DATA_PLANE_URL];[RSClient getInstance:WRITE_KEY config:[builder build]];
RSClient
is accessible after the initialization by [RSClient sharedInstance]
.let builder: RSConfigBuilder = RSConfigBuilder() .withDataPlaneUrl(DATA_PLANE_URL)RSClient.getInstance(WRITE_KEY, config: builder.build())
RSClient
is accesible after the initialization by RSClient.sharedInstance()
We automatically track the following optional events:
Application Installed
Application Updated
Application Opened
Application Backgrounded
withTrackLifecycleEvents
method of RSConfigBuilder
and passing false
. However, it is highly recommended to keep them enabled.RudderStack supports all the major API calls across all iOS devices via the SDK. These include the track
, identify
, and screen
calls.
Enabling/disabling user tracking via the optOut API (GDPR support)
RudderStack gives the users (e.g., an EU user) the ability to opt out of tracking any user activity until the user gives their consent. You can do this by leveraging RudderStack's optOut
API.
The optOut
API takes YES
or NO
as a Boolean value to enable or disable tracking user activities. This flag persists across device reboots.
The following snippet highlights the use of the optOut
API to disable user tracking:
[[RSClient sharedInstance] optOut:YES];
RSClient.sharedInstance()?.optOut(true)
Once the user grants their consent, you can enable user tracking once again by using the optOut
API with NO
or false
as a parameter sent to it, as shown:
[[RSClient sharedInstance] optOut:NO];
RSClient.sharedInstance()?.optOut(false)
The optOut
API is available in the RudderStack iOS SDK starting from version 1.0.24
.
Chromecast
Google Chromecast is a device that plugs into your TV or monitor with an HDMI port, and can be used to stream content from your phone or computer.
RudderStack supports integrating the iOS SDK with your Cast app. Follow these instructions to build your iOS sender app. Then, add the iOS SDK to it.
Follow the Google Cast developer guide for more details.
Track
You can record the users' activity through the track
method. Every user action is called as an event.
A sample track
event is as shown:
[[RSClient sharedInstance] track:@"simple_track_with_props" properties:@{ @"key_1" : @"value_1", @"key_2" : @"value_2"}];
RSClient.sharedInstance()?.track("test_user_id", properties: [ "key_1": "value_1", "key_2": "value_2"])
The track
method accepts the following parameters:
Name | Data Type | Required | Description |
---|---|---|---|
eventName | NSString | Yes | Name of the event you want to track |
properties | NSDictionary | No | Extra data properties you want to send along with the event |
options | RudderOption | No | Extra event options |
Identify
We capture deviceId
and use that as anonymousId
for identifying the user. This helps in tracking the users across the application installation.
To attach more information to the user, you can use the identify
method. Once you identify the user, the SDK persists all the user information and passes it to the subsequent track
or screen
calls. To reset the user identification, you can use the reset
method.
According to the Apple documentation, if the device has multiple apps from the same vendors, all those apps will be assigned the same deviceId
. If all the applications from a vendor are uninstalled, then on next install the app will be assigned a new deviceId
.
An example identify
event is as shown:
[[RSClient sharedInstance] identify:@"test_user_id"traits:@{@"foo": @"bar", @"foo1": @"bar1", @"email": @"test@gmail.com", @"key_1" : @"value_1", @"key_2" : @"value_2"}];
RSClient.sharedInstance()?.identify("test_user_id", traits: [ "key_1": "value_1", "key_2": "value_2", "email": "test@gmail.com"])
The identify
method accepts the following parameters:
Name | Data Type | Required | Description |
---|---|---|---|
userId | NSString | Yes | Developer identity for the user. |
traits | NSDictionary | No | Traits information for user. Use dict method of RudderTraits to convert to NSDictionary easily |
options | RudderOption | No | Extra options for the identify event. |
Screen
You can use the screen
call to record whenever the user sees a screen on the mobile device. You can also send some extra properties along with this event.
An example of the screen
event is as shown:
[[RSClient sharedInstance] screen:@"ViewController"];
RSClient.sharedInstance()?.screen("ViewController")
The screen
method accepts the following parameters:
Name | Data Type | Required | Description |
---|---|---|---|
screenName | NSString | Yes | Name of the screen viewed by the user. |
properties | NSDictionary | No | Extra property object that you want to pass along with the screen call. |
options | RudderOption | No | Extra options to be passed along with the screen event. |
Group
The group
call associates a user to a specific organization. A sample group
call for the API is below:
[[RSClient sharedInstance] group:@"sample_group_id" traits:@{@"foo": @"bar", @"foo1": @"bar1", @"email": @"ruchira@gmail.com"}];
RSClient.sharedInstance()?.group("test_group_id", traits: [ "key_1": "value_1", "key_2": "value_2"])
Alternatively, you can use the following method signature
Name | Data Type | Required | Description |
---|---|---|---|
groupId | String | Yes | An ID of the organization with which you want to associate your user |
traits | NSDictionary | No | Any other property of the organization you want to pass along with the call |
options | RudderOption | No | Event level options |
RudderStack does not persist the traits for the group across the sessions.
Alias
The alias
call associates the user with a new identification. A sample alias
call for the API is below:
[[RSClient sharedInstance] alias:@"new_user_id"];
RSClient.sharedInstance()?.alias("new_user_id")
Alternatively, you can use the following method signature
Name | Data Type | Required | Description |
---|---|---|---|
newId | String | Yes | The new userId you want to assign to the user |
options | RudderOption | No | Event level option |
We replace the old userId
with the newUserId
and we persist that identification across the sessions.
Reset
You can use the reset
method to clear the persisted traits
for the identify
call. This is required for the Logout
operations.
[[RSClient sharedInstance] reset];
RSClient.sharedInstance()?.reset()
Configuring the RudderStack client
You can configure your client based on the following parameters using RudderConfigBuilder
:
Parameter | Type | Description | Default Value |
---|---|---|---|
logLevel | int | Controls how much of the log you want to see from the SDK. | RSLogLevelNone |
dataPlaneUrl | string | Your Data Plane URL. | https://hosted.rudderlabs.com |
flushQueueSize | int | Number of events in a batch request sent to the server. | 30 |
dbThresholdCount | int | Number of events to be saved in the SQLite database. Once the limit is reached, older events are deleted from the DB. | 10000 |
sleepTimeout | int | Minimum waiting time to flush the events to the server. . | 10 seconds |
configRefreshInterval | int | Fetches the config from dashboard after the specified time. | 2 hours |
trackLifecycleEvents | boolean | Specify whether the SDK will capture application life cycle events automatically. | true |
recordScreenViews | boolean | Specify whether the SDK will capture screen view events automatically. | false |
enableBackgroundMode | boolean | Specify whether the SDK should send the events for some time when the app is moved to the background. Currently it is available only for iOS & tvOS . | false |
controlPlaneUrl | string | This parameter should be changed only if you are self-hosting the control plane. Check the Self-hosted control plane section below for more information. The iOS SDK will add /sourceConfig along with this URL to fetch the required configuration. | https://api.rudderlabs.com |
Self-hosted control plane
If you are using a device mode destination like Adjust, Firebase, etc., the iOS SDK needs to fetch the required configuration from the control plane. If you are using the Control Plane Lite utility to host your own control plane, then follow this guide and specify controlPlaneUrl
in RudderConfigBuilder
that points to your hosted source configuration file.
You shouldn't pass the controlPlaneUrl
parameter during SDK initialization if you are using RudderStack Cloud. This parameter is supported only if you are using our open-source Control Plane Lite utility to self-host your control plane.
Setting the device token
You can pass your device-token
for Push Notifications to be passed to the destinations which support Push Notification. We set the token
under context.device.token
.
Follow the instructions below:
[RSClient putDeviceToken:@"your_device_token"];
Advertisement ID
We have kept IDFA collection completely separate from the Core library so that the developer has better control over the same. You can pass the IDFA to putAdvertisementId
method to set it under context.device.advertisingId
Follow the instructions below:
#import <AdSupport/ASIdentifierManager.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions{ // Override point for customization after application launch. RSConfigBuilder *builder = [[RSConfigBuilder alloc] init]; [builder withDataPlaneURL:[[NSURL alloc] initWithString:DATA_PLANE_URL]]; [RSClient getInstance:WRITE_KEY config:[builder build]];
[[[RSClient sharedInstance] getContext] putAdvertisementId:[self getIDFA]];
return YES;}
- (NSString*) getIDFA { return [[[ASIdentifierManager sharedManager] advertisingIdentifier] UUIDString];}
ATTrackingManager authorization consent
You can pass the ATTrackingManager.trackingAuthorizationStatus to RudderStack and we'll pass it along to the relevant destinations wherever needed. For example AppsFlyer accepts this parameter for the attribution to work in their S2S mode.
Follow the instructions below:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions{ // Override point for customization after application launch. RSConfigBuilder *builder = [[RSConfigBuilder alloc] init]; [builder withDataPlaneURL:[[NSURL alloc] initWithString:DATA_PLANE_URL]]; [RSClient getInstance:WRITE_KEY config:[builder build]];
[[[RSClient sharedInstance] getContext] putAppTrackingConsent:RSATTAuthorize];
return YES;}
Following are the available options you can pass to the putAppTrackingConsent
method.
RSATTNotDetermined
RSATTRestricted
RSATTDenied
RSATTAuthorize
Anonymous ID
We use the deviceId
as anonymousId
by default. You can use the following method to override and use your own anonymousId
with the SDK.
An example of setting the anonymousId
is shown below:
[RSClient putAnonymousId:<ANONYMOUS_ID>];
Filtering events
When sending events to a destination via the device mode, you can explicitly specify which events should be discarded or allowed to flow through - by allowlisting or denylisting them.
Refer to the Client-side Event Filtering guide for more information on this feature.
Enabling/disabling events for specific destinations
The RudderStack iOS SDK allows you to enable or disable event flow to a specific destination or all the destinations to which the source is connected. You can specify these destinations by creating a RSOption
object as shown:
RSOption *option = [[RSOption alloc]init];//default value for `All` is true[option putIntegration:@"All" isEnabled:YES];// specifying destination by its display name[option putIntegration:@"Amplitude" isEnabled:YES];[option putIntegration:@"<destination display name>" isEnabled:<BOOL>];// specifying destination by its Factory instance[option putIntegrationWithFactory:[RudderMoengageFactory instance] isEnabled:NO];[option putIntegrationWithFactory:[<RudderIntegrationFactory> instance] isEnabled:<BOOL>];
let option:RSOption = RSOption();//default value for `All` is trueoption.putIntegration("All", isEnabled:true)// specifying destination by its display nameoption.putIntegration("Amplitude", isEnabled:true)option.putIntegration(<DESTINATION DISPLAY NAME>, isEnabled:<BOOL>)// specifying destination by its Factory instanceoption.putIntegration(with: RudderMoengageFactory.instance(), isEnabled: true);option.putIntegration(with: <RudderIntegrationFactory>.instance(), isEnabled:<BOOL>);
The keyword All
in the above snippet represents all the destinations the source is connected to. Its value is set to true
by default.
Make sure the destination display name
you pass while specifying the custom destinations should exactly match the destination name as shown here.
You can pass the destination(s) specified in the above snippet to the SDK in two ways:
1. Passing destinations while initializing the SDK
This is helpful when you want to enable/disable sending the events across all the event calls made using the SDK to the specified destinations.
RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withDataPlaneURL:[[NSURL alloc] initWithString:DATA_PLANE_URL]];[builder withLoglevel:RSLogLevelDebug];[builder withTrackLifecycleEvens:YES];[builder withRecordScreenViews:YES;[RSClient getInstance:WRITE_KEY config:[builder build] options:option]; // passing the rudderoption object containing the list of destinations you specified
let builder: RSConfigBuilder = RSConfigBuilder() .withLoglevel(RSLogLevelDebug) .withDataPlaneUrl(DATA_PLANE_URL) .withTrackLifecycleEvens(true) .withRecordScreenViews(true)RSClient.getInstance(WRITE_KEY, config: builder.build(),options: option)// passing the rudderoption object containing the list of destination(s) you specified
2. Passing destinations while making event calls
This approach is helpful when you want to enable/disable sending only a particular event to the specified destination(s) or if you want to override the specified destinations passed with the SDK initialization for a particular event.
[[RSClient sharedInstance] track:@"simple_track_with_props" properties:@{ @"key_1" : @"value_1", @"key_2" : @"value_2" } options:option]; // passing the rudderoption object containing the list of destination(s) you specified
let rudder: RSClient? = RSClient.sharedInstance()rudder?.track("track_with_props", properties: [ "key_1": "value_1", "key_2": "value_2", ],options:option) // passing the rudderoption object containing the list of destination(s) you specified
If you specify the destinations both while initializing the SDK as well as making an event call, then the destinations specified at the event level only will be considered.
External ID
You can pass your custom userId
along with standard userId
in your identify
calls. We add those values under context.externalId
. The following code snippet shows a way to add externalId
to your identify
request.
RSOption *identifyOptions = [[RSOption alloc] init];[identifyOptions putExternalId:@"brazeExternalId" withId:@"some_external_id_1"];[[RSClient sharedInstance] identify:@"testUserId" traits:@{@"firstname": @"First Name"} options:identifyOptions];
Debugging
If you run into any issues regarding the RudderStack iOS SDK, you can turn on the VERBOSE
or DEBUG
logging to find out what the issue is. To turn on the logging, change your RudderClient
initialization to the following:
RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withDataPlaneUrl:DATA_PLANE_URL];[builder withLoglevel:RudderLogLevelDebug];[RSClient getInstance:WRITE_KEY config:[builder build]];
let builder: RSConfigBuilder = RSConfigBuilder()builder.withDataPlaneUrl(<DATA_PLANE_URL>)builder.withLoglevel(RudderLogLevelDebug)RSClient.getInstance(<WRITE_KEY>, config: builder.build())
Developing a device mode destination
You can easily develop a device mode destination in case RudderStack doesn't support it already. Follow the steps listed in this section to do so.
More information on the RudderStack device mode can be found in the RudderStack Connection Modes guide.
- Create a
CustomFactory.h
file by extendingRSIntegrationFactory
, as shown:
#import <Foundation/Foundation.h>#import <Rudder/Rudder.h>
NS_ASSUME_NONNULL_BEGIN
@interface CustomFactory : NSObject<RSIntegrationFactory>
+ (instancetype) instance;
@end
NS_ASSUME_NONNULL_END
- Then, create a
CustomFactory.m
file, as shown:
#import <Foundation/Foundation.h>#import <Rudder/Rudder.h>#import "CustomFactory.h"#import "CustomIntegration.h"
@implementation CustomFactory
+ (instancetype)instance { static CustomFactory *sharedInstance; static dispatch_once_t onceToken; dispatch_once(&onceToken, ^{ sharedInstance = [[self alloc] init]; }); return sharedInstance;}
- (instancetype)init{ self = [super init]; return self;}
- (nonnull NSString *)key { return @"Custom Factory";}
- (nonnull id<RSIntegration>)initiate:(NSDictionary *)config client:(nonnull RSClient *)client rudderConfig:(nonnull RSConfig *)rudderConfig { return [[CustomIntegration alloc] initWithConfig:config withAnalytics:client];}
@end
- Next, create a
CustomIntegration.h
file by extendingRSIntegration
.
#import <Foundation/Foundation.h>#import <Rudder/Rudder.h>
NS_ASSUME_NONNULL_BEGIN
@interface CustomIntegration : NSObject<RSIntegration>
@property (nonatomic, strong) NSDictionary *config;@property (nonatomic, strong) RSClient *client;
- (instancetype)initWithConfig:(NSDictionary *)config withAnalytics:(RSClient *)client;
@end
NS_ASSUME_NONNULL_END
- Next, create a
CustomIntegration.m
file.
#import <Foundation/Foundation.h>#import <Rudder/Rudder.h>#import "CustomIntegration.h"
@implementation CustomIntegration
- (instancetype) initWithConfig:(NSDictionary *)config withAnalytics:(RSClient *)client { if (self == [super init]) { } return self;}
- (void) processRuderEvent:(nonnull RSMessage *)message { NSString *type = message.type; if ([type isEqualToString:@"identify"]) {// Do something } else if ([type isEqualToString:@"track"]) {// Do something } else if ([type isEqualToString:@"screen"]) {// Do something } else { [RSLogger logWarn:@"MessageType is not supported"]; }}
- (void) dump:(nonnull RSMessage *)message { [self processRuderEvent:message];}
- (void) reset {}
- (void) flush {}
@end
- Register the
CustomFactory
with the RudderStack iOS SDK during its initialization, as shown:
RSConfigBuilder *builder = [[RSConfigBuilder alloc] init]; [builder withDataPlaneURL:[[NSURL alloc] initWithString:DATA_PLANE_URL]]; [builder withLoglevel:RSLogLevelDebug]; [builder withTrackLifecycleEvens:NO]; [builder withRecordScreenViews:NO]; [builder withCustomFactory:[CustomFactory instance]]; [RSClient getInstance:WRITE_KEY config:[builder build]];
Some pointers to keep in mind:
- RudderStack's iOS SDK dumps every event it receives to the
dump()
method of theCustomFIntegration
class. From here, you can process the event and hand it over to the native SDK of the Device Mode destination. - The SDK also triggers the
reset()
method of theCustomFactory
class on everyreset()
call made via the SDK. You can use this to handle the destination-specific reset logic. - Make sure you do not duplicate the value of
KEY
present insideCustomFactory
, across multipleCustomFactory
that you develop.
flush
API
The iOS SDK supports the flush()
API. RudderStack retrieves all the messages present in the database, divides them into individual batches based on the specified queue size, and flushes them to the RudderStack server/backend.
For example, if the flushQueueSize
is 30 and there are 180 events in the database when the flush()
API is called, the SDK will retrieve all those events and divide them into batches of 30 messages each, that is, into 6 batches.
If a batch fails for some reason, RudderStack drops the remaining batches to maintain the sequence of the messages. A batch is considered as failed if it isn’t sent to the RudderStack server after 3 retries.
In the device mode, the flush()
API also calls the destination SDK’s flush()
API (if applicable).
For every flush()
call made via the iOS SDK, the flush()
method of the CustomFactory
class is also triggered, which can be used to handle the destination-specific reset logic. You can make a flush
call using the SDK as shown:
[[RSClient sharedInstance] flush];
FAQs
I'm facing issues building with Carthage on XCode 12. What should I do?
If you're facing an issue with Carthage and XCode 12, you can follow this workaround suggested by the Carthage team.
Does the SDK support tvOS ?
As of version 1.1.0
, the iOS SDK supports the tvOS platform.
Does the SDK support watchOS ?
As of version 1.3.0
, the iOS SDK supports the watchOS platform.
How do I migrate from v1.0.2?
Update the usage of the following classes as per the table below:
Previous Name | Updated Name |
---|---|
RudderClient | RSClient |
RudderConfig | RSConfig |
RudderConfigBuilder | RSConfigBuilder |
RudderLogLevelDebug |
Other |
How do I ensure the events tracked just before closing/backgrounding the app are sent immediately and not on the next app launch?
To ensure that the events tracked just before closing/backgrounding the app are sent to RudderStack immediately, you can set withEnableBackgroundMode
to YES
while creating the RSConfigBuilder
object as shown below:
Currently, this feature is available only for iOS
& tvOS
platforms.
RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withEnableBackgroundMode:YES];[RSClient getInstance:WRITE_KEY config:[builder build] options:defaultOption];
By doing so, your app requests iOS for some additional background run time to run the app, which in turn allows the SDK to immediately send the events tracked just before the app is closed/backgrounded, instead of waiting till the next app launch.
This SDK feature relies on the background mode capability offered by the iOS. There is no set number on the background run time the apps get, as it is completely abstracted by iOS. For more information, refer to this guide.
How can I get the user traits
after making the identify
call?
You can get the user traits after making an identify
call in the following way:
let traits = RSClient.sharedInstance()?.getContext().traits
NSDictionary* traits = [[RSClient sharedInstance] getContext].traits;
How does the SDK handle different client/server errors?
In case of client-side errors, e.g. if the source write key passed to the SDK is incorrect, RudderStack gives you a 400 Bad Request response and aborts the operation immediately. For other types of network errors (e.g. Invalid Data Plane URL), the SDK tries to flush the events to RudderStack in an incremental manner (every 1 second, 2 seconds, 3 seconds, and so on).
Why is there a larger difference between timestamp
and received_at
for iOS events vs. Android events?
This scenario is most likely caused by the default behavior of iOS apps staying open in the background for a shorter period of time after a user closes them.
When a user closes an iOS or Android app, events will still continue to be sent from the queue until the app closes in the background as well. Any events still in the queue will remain there until the user reopens the app. Due to this lag, there are some scenarios where there can be significant differences between timestamp
(when the event was created) and received_at
(when RudderStack actually receives the events).
For Android apps, events can be sent from the background after apps close for a longer period of time than iOS apps, therefore, more of the events coming from the Android SDK have closer timestamp
and received_at
times.
Does RudderStack integrate with SKAdNetwork?
RudderStack does not integrate with SKAdNetwork. However, SKAdNetwork can be directly integrated into an iOS application alongside RudderStack.
Can I disable event tracking until the user gives their consent?
Yes, you can.
RudderStack gives you the ability to disable tracking any user activity until the user gives their consent, by leveraging the optOut
API. This is required in cases where your app is audience-dependent (e.g. minors) or where you're using the app to track the user events (e.g. EU users) to meet the data protection and privacy regulations.
The optOut
API takes true
/ false
(in case of Swift) or YES
/ NO
(in case of Objective-C) as a value to enable or disable tracking user activities. So, to disable user tracking, you can use the optOut
API as shown:
[[RSClient sharedInstance] optOut:YES];
RSClient.sharedInstance()?.optOut(true)
Once the user gives their consent, you can enable user tracking again, as shown:
[[RSClient sharedInstance] optOut:NO];
RSClient.sharedInstance()?.optOut(false)
For more information on the optOut
API, refer to the Enabling/Disabling User Tracking via optOut API (GDPR Support) section above.
You only need to call the optOut
API with the required parameter only once, as the information persists within the device even if you reboot it.
Contact us
For any queries on any of the sections covered in this guide, you can contact us, or start a conversation in our Slack community.
If you come across any issues while using the iOS SDK, you can also open a new issue on our GitHub Issues page.